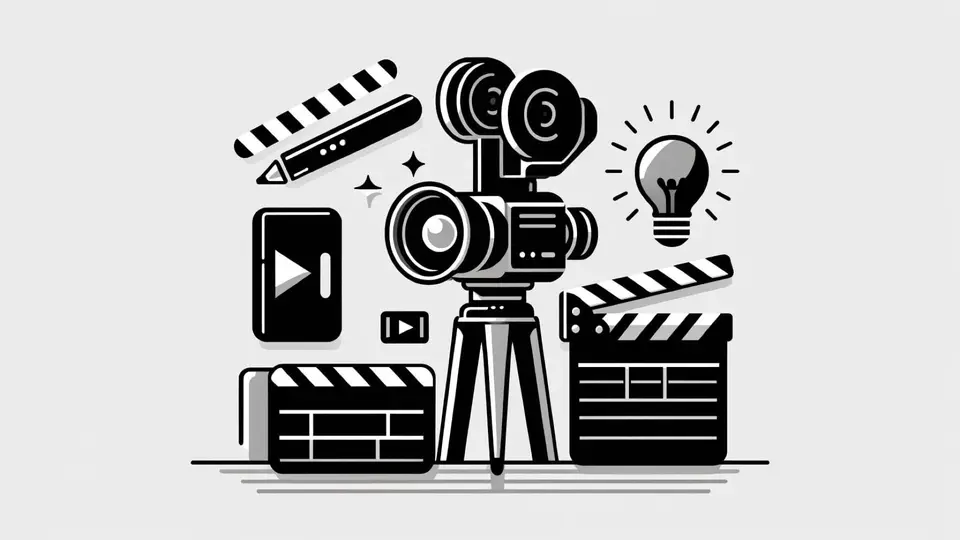
Content Creation
Selvin Ortiz
PHP continues to enhance its efficiency, readability, and overall capability.
However, some of these features remain under the radar for many developers. In this post, we'll explore 7 such underutilized features in modern PHP that can significantly improve your coding game.
Generators provide an easy way to implement simple iterators.
They allow you to iterate over data without needing to create an array in memory, which can be a significant memory saver.
function getLogLines($file) {
$handle = fopen($file, 'r');
while (!feof($handle)) {
yield fgets($handle);
}
fclose($handle);
}
The null coalescing operator (??) is a syntactic sugar that helps in returning the first operand if it exists and is not null; otherwise, it returns the second operand.
$username = $_GET['user'] ?? 'nobody';
echo $username;
function compare($a, $b) {
return $a <=> $b;
}
The Spaceship Operator (<=>) simplifies comparisons. It's a three-way comparison operator and is excellent for sorting.
Since PHP 7.4, you can now declare types for class properties. This ensures type safety, making your code more robust and self-documenting.
class User {
public int $id;
public string $name;
}
Anonymous classes are excellent for short-lived, single-use classes.
They can be as full-featured as any regular class but can be defined and instantiated on the fly.
$newClass = new class {
public function sayHello() {
echo "Hello, World!";
}
};
// Usage example
$newClass->sayHello();
Introduced in PHP 8.0, attributes provide a way to add metadata to classes, methods, or properties in a structured way.
This feature can be especially useful for frameworks.
#[Route('/api/posts/{id}', methods: ['GET'])]
class PostController {
// ...
}
Introduced in PHP 7.4, Weak References offer a way to retain a reference to an object without preventing its destruction. This feature is particularly useful in managing memory and avoiding memory leaks in applications that require large or complex object graphs.
What are Weak References? A Weak Reference allows the programmer to retain a reference to an object while not influencing its garbage collection lifecycle. Unlike regular references, which create a strong link to the object, weak references allow the object they reference to be garbage-collected if there are no other strong references to it.
The WeakReference class is used to create weak references. Here’s a simple example:
$obj = new stdClass;
$weakRef = WeakReference::create($obj);
// $obj can be garbage collected even if $weakRef still exists
unset($obj);
if ($weakRef->get()) {
// object still exists
} else {
// object has been destroyed
}
These features, though often underutilized, can greatly enhance the efficiency, readability, and functionality of your PHP code. Embracing them can lead to cleaner, more maintainable, and more expressive codebases.
Selvin Ortiz👋
I'm a software engineer and content creator.
I help brands develop software and content strategies 🚀
On this blog, I write about software development, emerging technology, technical leadership, and content creation ✨
Content Creation
Tech Tips
Tech Reviews
Professional Career
Cybersecurity
Web Development