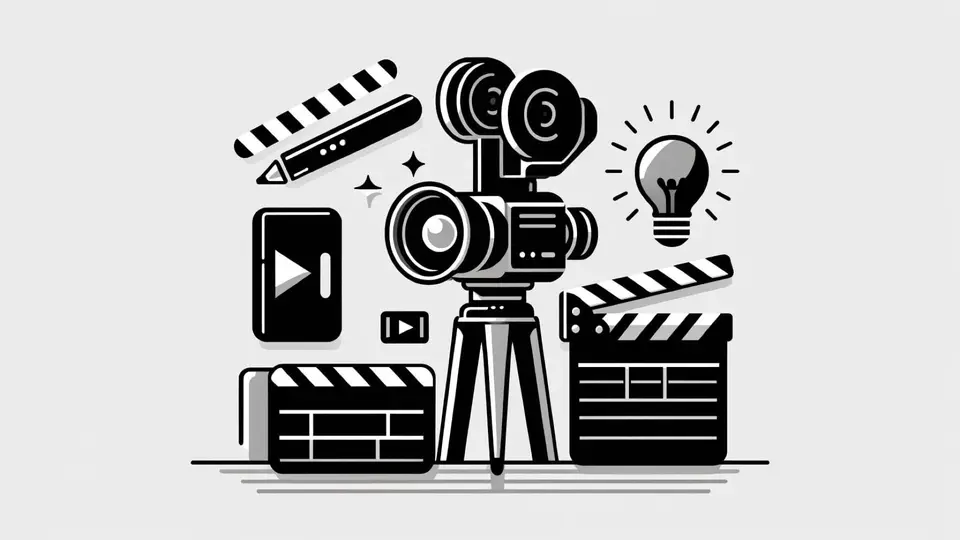
Content Creation
Selvin Ortiz
Most people who write code are not good copywriters, which is why most code comments in the wild are poorly written.
Taking the time to write clear, concise, and effective comments is a vital part of being a professional developer.
That said, many of you will disagree with my philosophy that by default, I don't write code comments.
I push my code to communicate my intent as far as I can before I reach for a comment.
A good code comment has three essential parts:
Context: What is the comment explaining? Is it describing a function, a variable, or a block of code? Provide the necessary context to understand what the comment is about.
Purpose: Why does this code exist? What problem does it solve? What is its intent? A good comment should explain the rationale behind the code, not just reiterate what the code is doing.
Clarity: Use simple, straightforward language. Avoid jargon, abbreviations, and ambiguity. The comment should be understandable to anyone reading the code, not just those familiar with the codebase.
// This helper function implements the exponential backoff with jitter strategy
// to retry a given operation with increasing delays between retries, up to a
// maximum number of attempts. It is useful for handling intermittent errors
// or transient network issues.
func retryWithBackoff(operation func() error, maxAttempts int) error {
// ...
}
// This regular expression pattern matches email addresses with specific requirements
// to ensure better validation than the standard filter_var() function.
$emailPattern = '/^(?!(?:(?:\x22?\x5C[\x00-\x7E]\x22?)|(?:\x22?[^\x5C\x22]\x22?)){255,})(?!(?:(?:\x22?\x5C[\x00-\x7E]\x22?)|(?:\x22?[^\x5C\x22]\x22?)){65,}@)(?:[\x21\x23-\x27\x2A\x2B\x2D\x2F-\x39\x3D\x3F\x5E-\x7E]+)(?:\.(?:[\x21\x23-\x27\x2A\x2B\x2D\x2F-\x39\x3D\x3F\x5E-\x7E]+))*@(?:(?:(?!.*[^.]{64,})(?:(?:(?:xn--)?[a-z0-9]+(?:-+[a-z0-9]+)*\.){1,126}){1,}(?:(?:[a-z][a-z0-9]*)|(?:(?:xn--)[a-z0-9]+))(?:-+[a-z0-9]+)*)|(?:\[(?:(?:IPv6:(?:(?:[a-f0-9]{1,4}(?::[a-f0-9]{1,4}){7})|(?:(?!(?:.*[a-f0-9][:\]]){7,})(?:[a-f0-9]{1,4}(?::[a-f0-9]{1,4}){0,5})?::(?:[a-f0-9]{1,4}(?::[a-f0-9]{1,4}){0,5})?)))|(?:(?:IPv6:(?:(?:[a-f0-
/**
* Calculates the total cost of a shopping cart, taking into account
* any applicable discounts and taxes.
*
* @param {Object[]} items - An array of items in the shopping cart
* @param {number} discountPercentage - The discount percentage to apply
* @param {number} taxRate - The tax rate to apply
* @returns {number} The total cost of the shopping cart
*/
function calculateTotal(items, discountPercentage, taxRate) {
// ...
}
These comments provide context, purpose, and clarity.
Not every line of code needs a comment. In fact, over-commenting can make your code harder to read and maintain.
Here are some guidelines for when to write comments:
Even well-intentioned developers can make mistakes when writing comments.
Here are some common pitfalls to avoid:
Writing great comments is an essential skill for any developer. It takes practice and discipline, but the effort pays off in more readable, maintainable, and collaborative code.
Remember to focus on providing context, explaining the purpose, and using clear language. And don't be afraid to revisit and refine your comments as your code evolves – after all, good comments are living documentation that should grow and change with your codebase.
Selvin Ortiz👋
I'm a software engineer and content creator.
I help brands develop software and content strategies 🚀
On this blog, I write about software development, emerging technology, technical leadership, and content creation ✨
Content Creation
Tech Tips
Tech Reviews
Professional Career
Cybersecurity
Web Development