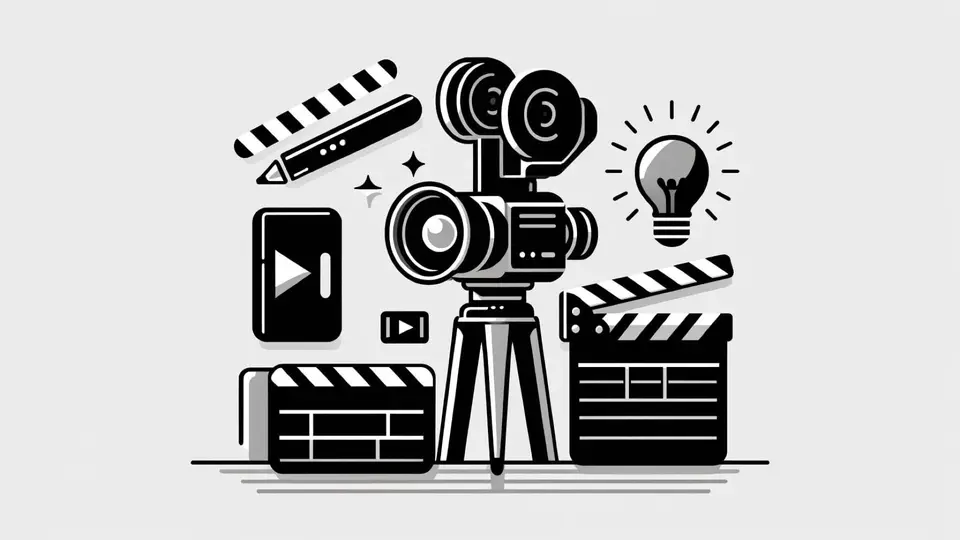
Content Creation
Selvin Ortiz
Today, I'm diving into a topic that's as confusing as it is unavoidable: working with timezones in Laravel. Anyone who's ever scheduled a meeting with colleagues across the globe or tried to coordinate a global product launch knows the drill.
Timezones can be a real headache.
Let's see how we can untangle this mess with practical solutions.
There are too many reasons to name but here is a short list.
Different systems follow different timezone standards, leading to confusion and mismatches.
This seasonal shift is the bane of scheduling. It varies by region and can throw off calculations.
Users often set their local time without specifying their timezone, leading to incorrect assumptions.
Storing and retrieving dates in a consistent timezone across a diverse user base is a complex task and often leads to time drift.
By the way, this is not an exhaustive list🤦‍♂️
Let's tackle these issues with some real-world examples and Laravel-centric solutions.
// Use Laravel's built-in Carbon library for consistent timezone handling
use Carbon\Carbon;
$userTimezone = 'America/New_York';
$meetingTime = Carbon::createFromFormat('Y-m-d H:i', '2024-01-17 15:00', $userTimezone);
echo $meetingTime->tz('UTC');
This snippet converts a user-specified time to UTC, ensuring a standardized format across different systems.
If you're using Laravel, you already have Carbon. If not, you can pull that in through composer.
// Carbon automatically adjusts for DST
$dstTime = Carbon::createFromDate(2024-03-10, $userTimezone);
echo $dstTime->addHours(24);
Carbon intelligently handles the DST transition, so you don’t have to worry about the 1-hour shift.
Encourage users to specify their timezone explicitly. Laravel can detect the user’s timezone based on IP, but it's better to have users confirm it.
Always store dates in UTC in your database. Convert them to the user's local timezone when displaying.
// Storing date in UTC
$utcDate = Carbon::now('UTC');
DB::table('events')->insert(['event_date' => $utcDate]);
// Retrieving and converting to user's timezone
$eventDate = DB::table('events')->first()->event_date;
echo Carbon::createFromFormat('Y-m-d H:i:s', $eventDate, 'UTC')->tz($userTimezone);
Ensure everyone understands the importance of consistent timezone handling.
Timezone issues often surface only in production. Test across different scenarios and watch out of time drifts.
Timezone rules can change. Keep your timezone data updated with tools like TimezoneDB.
Timezone management in PHP can be tricky, but with the right approach, it's manageable. Embrace tools like Carbon, enforce consistency in storing time data, and always be prepared for the quirks of DST.
By adhering to these practices, you'll reduce the headaches associated with timezone differences and ensure a smoother experience for both your team and your users.
Happy coding ✌️
Selvin Ortizđź‘‹
I'm a software engineer and content creator.
I help brands develop software and content strategies 🚀
On this blog, I write about software development, emerging technology, technical leadership, and content creation ✨
Content Creation
Tech Tips
Tech Reviews
Professional Career
Cybersecurity
Web Development