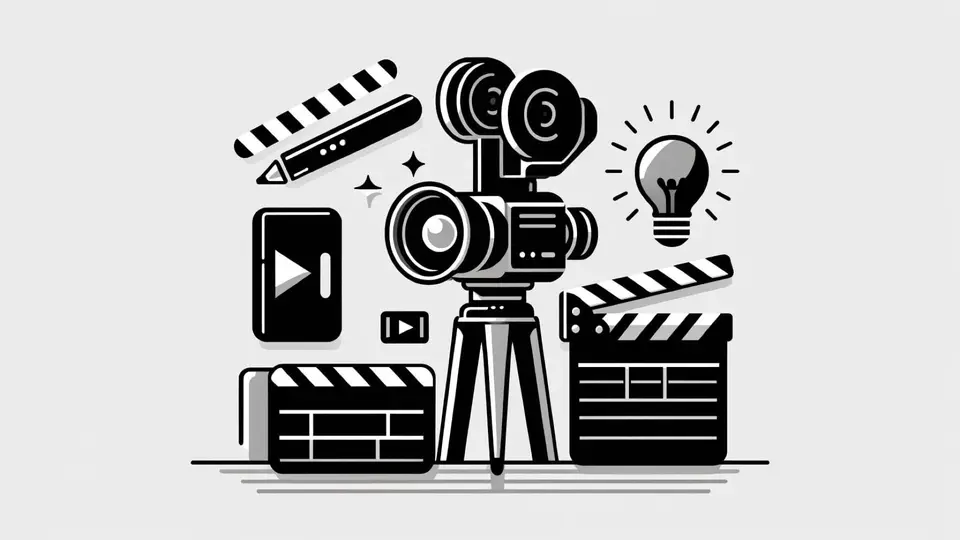
Content Creation
Selvin Ortiz
Traditionally, PHP uses square brackets for array access.
However, dot notation, a popular feature in many languages like JavaScript, can be implemented in PHP for a more intuitive and readable approach to array traversal.
Dot notation is a syntax for accessing the elements of an array or object properties using a dot (.
) as the separator. For example, object.property
in JavaScript. While PHP doesn't natively support dot notation for arrays, we can simulate this behavior.
To utilize dot notation in PHP, we create a function that translates dot-notated keys into standard PHP array keys.
Here's a basic implementation:
function getArrayValueByKey($array, $key, $default = null) {
$keys = explode('.', $key);
foreach ($keys as $k) {
if (!isset($array[$k])) {
return $default;
}
$array = $array[$k];
}
return $array;
}
// Usage example
$user = [
'name' => [
'first' => 'John',
'last' => 'Doe'
],
'contact' => [
'email' => '[email protected]'
]
];
// Example output
$firstName = getArrayValueByKey($user, 'name.first');
echo $firstName; // Outputs: John
Our solution above only covers single level nesting.
For a more robust solution, we can add the ability to fetch values with arbitrary levels of nesting.
function getArrayValueByKey($array, $key, $default = null) {
if (is_null($key)) {
return $default;
}
if (isset($array[$key])) {
return $array[$key];
}
foreach (explode('.', $key) as $segment) {
if (!is_array($array) || !array_key_exists($segment, $array)) {
return $default;
}
$array = $array[$segment];
}
return $array;
}
// Usage example
$array = [
'user' => [
'profile' => [
'name' => 'John Doe'
]
]
];
// Example output
$name = getArrayValueByKey($array, 'user.profile.name'); // Returns 'John Doe'
That's a little better.
However, for a full implementation, you should checkout https://github.com/adbario/php-dot-notation
Implementing dot notation in PHP for array traversal can significantly enhance code readability and maintainability. While it's not a native feature, with a simple function, you can achieve similar functionality, making your PHP code more elegant and accessible.
Selvin Ortizđź‘‹
I'm a software engineer and content creator.
I help brands develop software and content strategies 🚀
On this blog, I write about software development, emerging technology, technical leadership, and content creation ✨
Content Creation
Tech Tips
Tech Reviews
Professional Career
Cybersecurity
Web Development